app.component.ts
import { Component } from '@angular/core';
import {
NgbCalendar, NgbDate, NgbDatepicker, NgbDateStruct
} from '@ng-bootstrap/ng-bootstrap';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styles: [`
.custom-day {
text-align: center;
padding: 0.185rem 0.25rem;
display: inline-block;
height: 2rem;
width: 2rem;
}
.custom-day.focused {
background-color: Lavendar;
}
.custom-day.range, .custom-day:hover {
background-color: MidnightBlue;
color: white;
}
.custom-day.faded {
background-color: LightSteelBlue;
}
`]
})
export class AppComponent {
hoveredDate: NgbDate | null = null;
fromDate: NgbDate;
toDate: NgbDate | null = null;
constructor(private calendar: NgbCalendar) {
this.fromDate = calendar.getToday();
this.toDate = calendar.getNext(calendar.getToday(), 'd', 8);
}
onDateSelection(date: NgbDate) {
if (!this.fromDate && !this.toDate) {
this.fromDate = date;
} else if (this.fromDate && !this.toDate
&& date.after(this.fromDate)) {
this.toDate = date;
} else {
this.toDate = null;
this.fromDate = date;
}
}
isHovered(date: NgbDate) {
return this.fromDate && !this.toDate && this.hoveredDate
&& date.after(this.fromDate) && date.before(this.hoveredDate);
}
isInside(date: NgbDate) {
return this.toDate && date.after(this.fromDate)
&& date.before(this.toDate);
}
isRange(date: NgbDate) {
return date.equals(this.fromDate)
|| (this.toDate && date.equals(this.toDate))
|| this.isInside(date) || this.isHovered(date);
}
}
app.component.html
<h4 style="padding-top:25px; padding-bottom:25px;">
Angular Bootstrap - Select date range in a Datepicker
</h4>
<h5><b>Selected Date Range:</b></h5>
<h5>Form : {{ fromDate | json}}</h5>
<h5>To : {{ toDate | json}}</h5>
<br/>
<ngb-datepicker
#datePicker
(dateSelect)="onDateSelection($event)"
[displayMonths]="2"
[dayTemplate]="t"
outsideDays="collapsed">
</ngb-datepicker>
<ng-template #t let-date let-focused="focused">
<span class="custom-day"
[class.focused]="focused"
[class.range]="isRange(date)"
[class.faded]="isHovered(date) || isInside(date)"
(mouseenter)="hoveredDate = date"
(mouseleave)="hoveredDate = null">
{{ date.day }}
</span>
</ng-template>

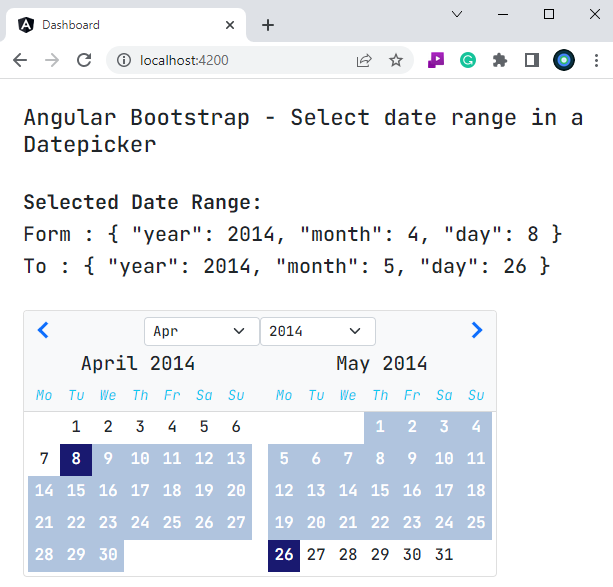
- angular bootstrap - Popover with HTML content
- angular bootstrap - How to delay Popover open close
- angular bootstrap - How to use Timepicker
- angular bootstrap - Timepicker with second
- angular bootstrap - How to use Datepicker
- angular bootstrap - Select today from Datepicker
- angular bootstrap - Datepicker navigate & select today
- angular bootstrap - Datepicker navigate event
- angular bootstrap - Show multiple months in a Datepicker
- angular bootstrap - Select date range from Datepicker popup
- angular bootstrap - How to enable disable Datepicker
- angular bootstrap - Datepicker custom day style
- angular bootstrap - How to use Accordion
- angular bootstrap - Open an Accordion panel at a time
- angular bootstrap - Toggle Accordion panel on button click