app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
randomNumberInt: number = -1;
generateRandomNumberInRange(min: number, max: number) {
// Minimum and maximum number both included
this.randomNumberInt = Math.floor(
Math.random() * (max - min + 1) + min
);
}
}
app.component.html
<h2>Angular - Random Number In Range</h2>
<ng-template [ngIf]="randomNumberInt>=0">
Random Number Int: {{randomNumberInt}}
</ng-template>
<br/><br/>
<button
(click)="generateRandomNumberInRange(0,10)"
style="padding:12px;">
Get Random Number
</button>

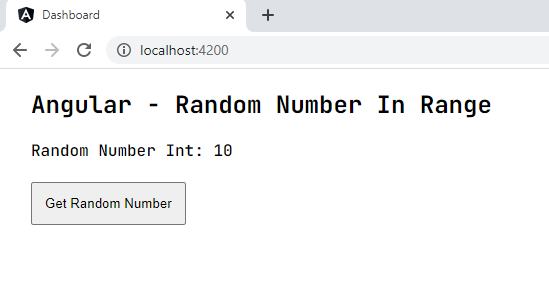
- angular - How to use ternary operator
- angular - How to generate random number
- angular - Generate random whole number
- angular - How to submit form data
- angular bootstrap - Dismissible alert
- angular bootstrap - Danger warning success alert
- angular bootstrap - Alert closed event
- angular bootstrap - Rating hover event
- angular bootstrap - Readonly & editable Rating
- angular bootstrap - How to show Toast message
- angular bootstrap - How to use Popover
- angular bootstrap - Open Popover on mouse hover
- angular bootstrap - Open close Popover on button click
- angular bootstrap - Timepicker without spinner
- angular bootstrap - Timepicker custom steps