app.component.ts
import { Component } from '@angular/core';
import { NgForm } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
formData:any = {};
getFormValue(data:NgForm){
this.formData = data.value;
}
}
app.component.html
<h2>Angular - Submit Form Data</h2>
<h3>
Submitted name: {{formData.firstName}} {{formData.lastName}}
age {{formData.age}}
</h3>
<form #userForm="ngForm" (submit)="getFormValue(userForm)">
<input
name="firstName"
type="input"
placeholder="First name"
style="padding:12px; font-size:large;"
ngModel
/>
<br/><br/>
<input
name="lastName"
type="input"
placeholder="Last name"
style="padding:12px; font-size:large;"
ngModel
/>
<br/><br/>
<input
name="age"
type="input"
placeholder="Age"
style="padding:12px; font-size:large;"
ngModel
/>
<br/><br/>
<button style="padding:12px;">Submit</button>
</form>
app.module.ts
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }

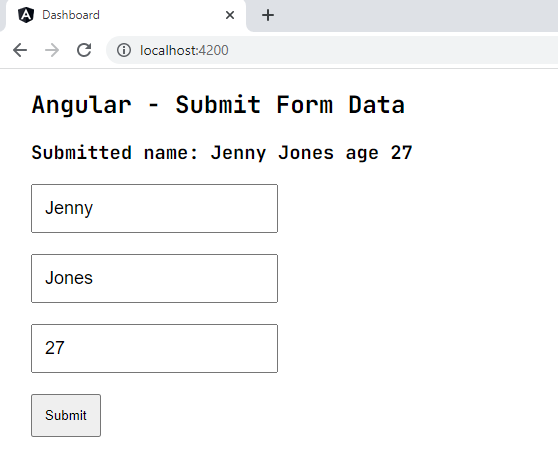
- angular - Interpolation JavaScript method
- angular - Interpolation call method
- angular - How to use if then condition
- angular - How to use if statement
- angular - Loop through an array of objects
- angular - How to use nested loop
- angular - How to use Button click event
- angular - How to call function on Button click
- angular - How to use ternary operator
- angular - How to generate random number
- angular - Generate random number in range
- angular - Generate random whole number
- angular bootstrap - Dismissible alert
- angular bootstrap - Danger warning success alert
- angular bootstrap - Alert closed event